206. Reverse Linked List
1) 문제 설명
Given the head of a singly linked list, reverse the list, and return the reversed list.
Example 1:
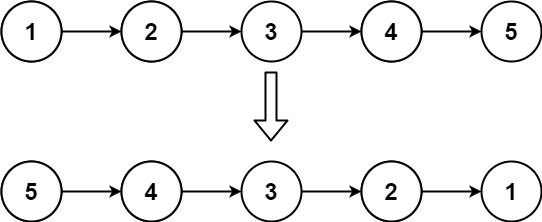
Input: head = [1,2,3,4,5]
Output: [5,4,3,2,1]
Example 2:
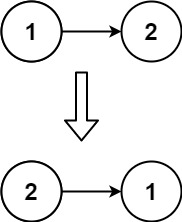
Input: head = [1,2]
Output: [2,1]
Example 3:
Input: head = []
Output: []
2) 제한 사항
- The number of nodes in the list is the range [0, 5000].
- -5000 <= Node.val <= 5000
3) 도전 과제
A linked list can be reversed either iteratively or recursively. Could you implement both?
4) 풀이
단방향 연결리스트의 값을 받아서 역순으로 기록하여 반환하였다.
간단한 문제이므로 다른 사람의 풀이 없이 넘어간다.
5) 소스 코드 및 결과
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
ListNode nextNode = null;
while (current != null) {
nextNode = current.next;
current.next = prev;
prev = current;
current = nextNode;
}
return prev;
}
}
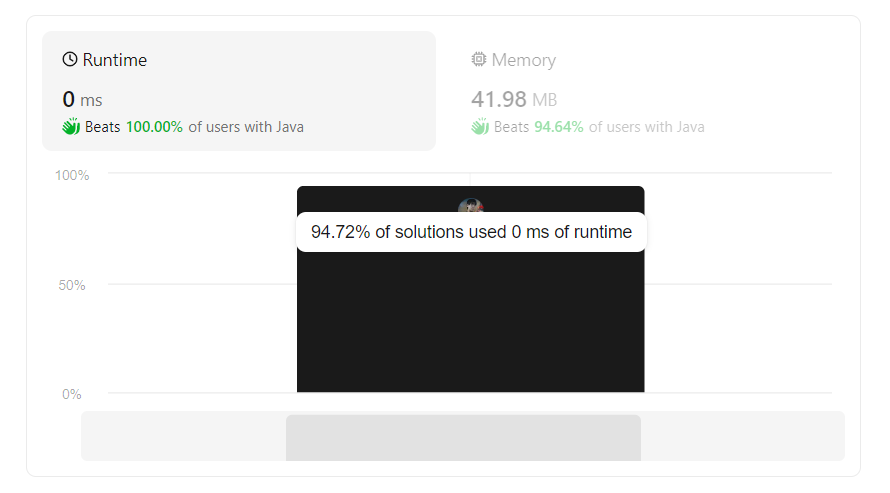
6) 다른 사람의 풀이
X
'알고리즘 풀이' 카테고리의 다른 글
240322 LeetCode 문제 풀이 (0) | 2024.03.22 |
---|---|
240314 LeetCode 문제 풀이 (1) | 2024.03.14 |
240313 LeetCode 문제 풀이 (0) | 2024.03.13 |
240311 LeetCode 문제 풀이 (0) | 2024.03.11 |
240308 LeetCode 문제 풀이 (0) | 2024.03.09 |